/* eslint-disable max-len */
/* eslint-disable linebreak-style */
/* eslint-disable no-undef */
/* eslint-disable operator-linebreak */
/* eslint-disable no-console */
/* eslint-disable camelcase */
var hasaudio
if ('speechSynthesis' in window) {
hasaudio = true
console.log("speech synthesis");
} else {
hasaudio = false
console.log("no speech synthesis");
}
speechSynthesis.cancel();
var sentences = []
var current_response;
var current_counter;
var wrong_counter;
var voices = []
setTimeout(() => {
voices = speechSynthesis.getVoices();
//console.log(voices)
voices.forEach(function(voice, i) {
console.log(voice.name);
});
}, 60);
const action_name = "action_greet_user";
//const rasa_server_url = "http://localhost:5005/webhooks/rest/webhook";
//const rasa_server_url = "http://localhost:5005/socketio";
//const rasa_server_url = "http://localhost:5005/webhooks/myio/webhook";
//const rasa_server_url = "http://127.0.0.1:5055/async";
const rasa_server_url = "/bot/ajax";
//const sender_id = uuidv4();
var sender_id = ""
var socket
//var mediaRecorder;
//var chunks = [];
//
//var AudioContext = window.AudioContext || window.webkitAudioContext;
//var audioContext; //audio context to help us record
//var recorder;
//
//if (navigator.mediaDevices.getUserMedia) {
// const constraints = { audio: true };
// navigator.mediaDevices.getUserMedia(constraints).then(
// stream => {
// console.log("授权成功!");
//
// audioContext = new AudioContext();
// var source = audioContext.createMediaStreamSource(stream);
// recorder = new Recorder(source, { sampleBits: 16, sampleRate: 16000, numChannels: 1 });
//
// //mediaRecorder = new MediaRecorder(stream);
//
// //mediaRecorder.ondataavailable = e => {
// // chunks.push(e.data);
// //};
//
// //mediaRecorder.onstop = e => {
// // var blob = new Blob(chunks, { type: "audio/ogg; codecs=opus" });
// // chunks = [];
// // var audioURL = window.URL.createObjectURL(blob);
//
// // new Audio(audioURL).play()
// //};
// },
// () => {
// console.error("授权失败!");
// }
// );
//} else {
// console.error("浏览器不支持 getUserMedia");
//}
// Bot pop-up intro
//document.addEventListener("DOMContentLoaded", () => {
// const elemsTap = document.querySelector(".tap-target");
// // eslint-disable-next-line no-undef
// const instancesTap = M.TapTarget.init(elemsTap, {});
// instancesTap.open();
// setTimeout(() => {
// instancesTap.close();
// }, 4000);
//});
// initialization
$(document).ready(() => {
// Bot pop-up intro
$("div").removeClass("tap-target-origin");
// drop down menu for close, restart conversation & clear the chats.
$(".dropdown-trigger").dropdown();
// initiate the modal for displaying the charts,
// if you dont have charts, then you comment the below line
$(".modal").modal();
$(".widget").show();
$(".profile_div").hide();
$(".usrInput").focus();
sender_id = document.getElementById("sid").innerHTML;
first_sen = document.getElementById("first_sen").innerHTML;
sentences = (document.getElementById("post_body").innerHTML || '').split(/[,,;,!,?,。]+/).map((item)=>item.trim());
const br = '
No information on ${category}.
`;
$(BotResponse).appendTo(".chats").hide().fadeIn(1000);
}
}
if (Object.hasOwnProperty.call(response[i], "uploadfile")) {
//const BotResponse = `
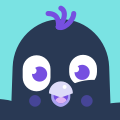
Pick up one .txt file and send
`;
const BotResponse = `
Pick up one .txt file and send
`;
$(BotResponse).appendTo(".chats").hide().fadeIn(1000);
document.querySelector('#uploadWords').addEventListener('change', event => {
handleUpload(event)
})
}
// check if the response contains "buttons"
if (Object.hasOwnProperty.call(response[i], "buttons")) {
if (response[i].buttons.length > 0) {
addSuggestion(response[i].buttons);
}
}
// check if the response contains "attachment"
if (Object.hasOwnProperty.call(response[i], "attachment")) {
if (response[i].attachment != null) {
if (response[i].attachment.type === "video") {
// check if the attachment type is "video"
const video_url = response[i].attachment.payload.src;
const BotResponse = `
`;
$(BotResponse).appendTo(".chats").hide().fadeIn(1000);
} else if (response[i].attachment.type === "audio") {
const audio_url = response[i].attachment.payload.src;
console.log("from bot audio");
new Audio(audio_url).play()
}
}
}
// check if the response contains "custom" message
if (Object.hasOwnProperty.call(response[i], "custom")) {
const { payload } = response[i].custom;
if (payload === "quickReplies") {
// check if the custom payload type is "quickReplies"
const quickRepliesData = response[i].custom.data;
showQuickReplies(quickRepliesData);
return;
}
// check if the custom payload type is "pdf_attachment"
if (payload === "pdf_attachment") {
renderPdfAttachment(response[i]);
return;
}
// check if the custom payload type is "dropDown"
if (payload === "dropDown") {
const dropDownData = response[i].custom.data;
renderDropDwon(dropDownData);
return;
}
// check if the custom payload type is "location"
if (payload === "location") {
$("#userInput").prop("disabled", true);
getLocation();
scrollToBottomOfResults();
return;
}
// check if the custom payload type is "cardsCarousel"
if (payload === "cardsCarousel") {
const restaurantsData = response[i].custom.data;
showCardsCarousel(restaurantsData);
return;
}
// check if the custom payload type is "chart"
if (payload === "chart") {
/**
* sample format of the charts data:
* var chartData = { "title": "Leaves", "labels": ["Sick Leave", "Casual Leave", "Earned Leave", "Flexi Leave"], "backgroundColor": ["#36a2eb", "#ffcd56", "#ff6384", "#009688", "#c45850"], "chartsData": [5, 10, 22, 3], "chartType": "pie", "displayLegend": "true" }
*/
const chartData = response[i].custom.data;
const {
title,
labels,
backgroundColor,
chartsData,
chartType,
displayLegend,
} = chartData;
// pass the above variable to createChart function
createChart(
title,
labels,
backgroundColor,
chartsData,
chartType,
displayLegend
);
// on click of expand button, render the chart in the charts modal
$(document).on("click", "#expand", () => {
createChartinModal(
title,
labels,
backgroundColor,
chartsData,
chartType,
displayLegend
);
});
return;
}
// check of the custom payload type is "collapsible"
if (payload === "collapsible") {
const { data } = response[i].custom;
// pass the data variable to createCollapsible function
createCollapsible(data);
}
}
}
scrollToBottomOfResults();
}
}, 500);
}
/**
* sends an event to the bot,
* so that bot can start the conversation by greeting the user
*
* `Note: this method will only work in Rasa 1.x`
*/
function actionTrigger() {
$.ajax({
url: `http://localhost:5005/conversations/${sender_id}/execute`,
type: "POST",
contentType: "application/json",
data: JSON.stringify({
name: action_name,
policy: "MappingPolicy",
confidence: "0.98",
}),
success(botResponse, status) {
console.log("Response from Rasa: ", botResponse, "\nStatus: ", status);
if (Object.hasOwnProperty.call(botResponse, "messages")) {
setBotResponse(botResponse.messages);
}
$("#userInput").prop("disabled", false);
},
error(xhr, textStatus) {
// if there is no response from rasa server
setBotResponse("");
console.log("Error from bot end: ", textStatus);
$("#userInput").prop("disabled", false);
},
});
}
/**
* sends an event to the custom action server,
* so that bot can start the conversation by greeting the user
*
* Make sure you run action server using the command
* `rasa run actions --cors "*"`
*
* `Note: this method will only work in Rasa 2.x`
*/
// eslint-disable-next-line no-unused-vars
function customActionTrigger() {
$.ajax({
url: "http://localhost:5055/webhook/",
type: "POST",
contentType: "application/json",
data: JSON.stringify({
next_action: action_name,
tracker: {
sender_id,
},
}),
success(botResponse, status) {
console.log("Response from Rasa: ", botResponse, "\nStatus: ", status);
if (Object.hasOwnProperty.call(botResponse, "responses")) {
setBotResponse(botResponse.responses);
}
$("#userInput").prop("disabled", false);
},
error(xhr, textStatus) {
// if there is no response from rasa server
setBotResponse("");
console.log("Error from bot end: ", textStatus);
$("#userInput").prop("disabled", false);
},
});
}
function getCookie(name) {
var r = document.cookie.match("\\b" + name + "=([^;]*)\\b");
return r ? r[1] : undefined;
}
function send(message) {
console.log(message)
//socket.emit('user_uttered', JSON.stringify({ message, sender: sender_id }))
socket.emit('user_uttered', JSON.stringify({ message }))
}
/**
* sends the user message to the rasa server,
* @param {String} message user message
*/
function sendAjax(message) {
$.ajax({
url: rasa_server_url,
type: "POST",
headers: { 'X-CSRFTOKEN': getCookie("csrftoken") }, // 这里是headers,不是data
contentType: "application/json",
data: JSON.stringify({ message, sender: sender_id }),
success(botResponse, status) {
console.log("Response from Rasa: ", botResponse, "\nStatus: ", status);
// if user wants to restart the chat and clear the existing chat contents
if (message.toLowerCase() === "/restart") {
$("#userInput").prop("disabled", false);
// if you want the bot to start the conversation after restart
// customActionTrigger();
return;
}
setBotResponse(botResponse);
},
error(xhr, textStatus) {
if (message.toLowerCase() === "/restart") {
$("#userInput").prop("disabled", false);
// if you want the bot to start the conversation after the restart action.
// actionTrigger();
// return;
}
// if there is no response from rasa server, set error bot response
setBotResponse("");
console.log("Error from bot end: ", textStatus);
},
});
}
/**
* clears the conversation from the chat screen
* & sends the `/resart` event to the Rasa server
*/
function restartConversation() {
$("#userInput").prop("disabled", true);
// destroy the existing chart
$(".collapsible").remove();
if (typeof chatChart !== "undefined") {
chatChart.destroy();
}
$(".chart-container").remove();
if (typeof modalChart !== "undefined") {
modalChart.destroy();
}
$(".chats").html("");
$(".usrInput").val("");
send("/restart");
}
// triggers restartConversation function.
$("#restart").click(() => {
restartConversation();
});
/**
* if user hits enter or send button
* */
$(".usrInput").on("keyup keypress", (e) => {
const keyCode = e.keyCode || e.which;
const text = $(".usrInput").val();
if (keyCode === 13) {
if (text === "" || $.trim(text) === "") {
e.preventDefault();
return false;
}
// destroy the existing chart, if yu are not using charts, then comment the below lines
$(".collapsible").remove();
$(".dropDownMsg").remove();
if (typeof chatChart !== "undefined") {
chatChart.destroy();
}
$(".chart-container").remove();
if (typeof modalChart !== "undefined") {
modalChart.destroy();
}
$("#paginated_cards").remove();
$(".suggestions").remove();
$(".quickReplies").remove();
$(".usrInput").blur();
setUserResponse(text);
send(text);
e.preventDefault();
return false;
}
return true;
});
function createLink(blob) {
var url = URL.createObjectURL(blob);
new Audio(url).play()
const request = new XMLHttpRequest();
request.open('GET', url, true);
request.responseType = 'blob';
request.onload = function() {
const reader = new FileReader();
reader.readAsDataURL(request.response);
reader.onload = function(el) {
// console.log('DataURL:', el.target.result);
console.log('SEND RESULT TO THE BOT');
send(el.target.result);
};
};
request.send();
}
function nextWord() {
if (current_counter + 1 >= sentences.length) {
current_counter = 0;
} else {
current_counter += 1;
}
current_response = sentences[current_counter];
message = {text: current_response};
setBotResponse([message]);
wrong_counter = 0;
}
$("#uploadFile").on("click", (e) => {
send('/uploadfile')
$(".usrInput").focus();
});
$("#nextWord").on("click", (e) => {
send('/nx')
$(".usrInput").focus();
});
$("#meaning").on("click", (e) => {
send('/mn')
$(".usrInput").focus();
});
$("#spell").on("click", (e) => {
send('/spell')
$(".usrInput").focus();
});
$("#mark").on("click", (e) => {
send('/mk')
$(".usrInput").focus();
});
$("#volume").on("click", (e) => {
var vv = document.getElementById("voli");
if (vv.title == "sound on") {
vv.className = "fa fa-volume-off"
vv.title = "sound off"
} else {
vv.className = "fa fa-volume-up"
vv.title = "sound on"
}
$(".usrInput").focus();
});
$("#repeatWord").on("click", (e) => {
//setBotResponse(current_response)
send('/rp')
$(".usrInput").focus();
});
$("#boltWord").on("click", (e) => {
send("/bolt");
$(".usrInput").val("");
$(".usrInput").focus();
scrollToBottomOfResults();
//showBotTyping();
e.preventDefault();
return false;
});
$("#sendButton").on("click", (e) => {
//if (recorder.recording) {
// recorder.stop();
// recorder.exportWAV(createLink);
// recorder.clear();
// console.log("stop record");
//} else {
// recorder.record();
// window.setTimeout(() => {
// if (recorder.recording) {
// recorder.stop();
// recorder.exportWAV(createLink);
// recorder.clear();
// console.log('10 seconds, stop recording');
// }
// }, 10000);
// console.log("recording...");
//}
////if (mediaRecorder.state === "recording") {
//// mediaRecorder.stop();
//// //recordBtn.textContent = "record";
//// console.log("录音结束");
////} else {
//// mediaRecorder.start();
//// console.log("录音中...");
//// //recordBtn.textContent = "stop";
////}
////console.log("录音器状态:", mediaRecorder.state);
const text = $(".usrInput").val();
if (text === "" || $.trim(text) === "") {
e.preventDefault();
return false;
}
// destroy the existing chart
if (typeof chatChart !== "undefined") {
chatChart.destroy();
}
$(".chart-container").remove();
if (typeof modalChart !== "undefined") {
modalChart.destroy();
}
$(".suggestions").remove();
$("#paginated_cards").remove();
$(".quickReplies").remove();
$(".usrInput").blur();
$(".dropDownMsg").remove();
setUserResponse(text);
send(text);
e.preventDefault();
return false;
});
// Toggle the chatbot screen
$("#profile_div").click(() => {
$(".profile_div").toggle();
$(".widget").toggle();
});
// on click of suggestion's button, get the title value and send it to rasa
$(document).on("click", ".menu .menuChips", function () {
const text = this.innerText;
const payload = this.getAttribute("data-payload");
console.log("payload: ", this.getAttribute("data-payload"));
setUserResponse(text);
// send(payload);
send(text);
// delete the suggestions once user click on it.
$(".suggestions").remove();
});
// clear function to clear the chat contents of the widget.
$("#clear").click(() => {
$(".chats").fadeOut("normal", () => {
$(".chats").html("");
$(".chats").fadeIn();
});
});
// close function to close the widget.
$("#close").click(() => {
$(".profile_div").toggle();
$(".widget").toggle();
scrollToBottomOfResults();
});
// on click of carousel cards, get the payload value and send it to rasa
$(document).on("click", ".cardTitle .deck", function () {
const text = this.innerText;
const cate = this.getAttribute("cate");
const payload = this.getAttribute("title");
cmd = "/"+cate+" "+payload;
setUserResponse(cmd)
send(cmd);
// delete the carsousel cards
$("#paginated_cards").remove();
});
// on click of quickreplies, get the payload value and send it to rasa
$(document).on("click", ".quickReplies .chip", function () {
const text = this.innerText;
const payload = this.getAttribute("data-payload");
console.log("chip payload: ", this.getAttribute("data-payload"));
setUserResponse(text);
send(payload);
// delete the quickreplies
$(".quickReplies").remove();
});
function speak(text) {
if (text == '') {
return
}
if (text.includes("XXX ")) {
var textes = text.split("XXX ");
speakExt(textes[0], 1.0);
speakExt(textes[1], 2.0);
} else {
speakExt(text, 1.0);
}
}
function isChinese(str) {
var pattern = new RegExp("[\u4E00-\u9FA5]+");
if(pattern.test(str)){
return true;
}
return false;
}
// Create a new utterance for the specified text and add it to
// the queue.
function speakExt(text, rate) {
// Create a new instance of SpeechSynthesisUtterance.
var msg = new SpeechSynthesisUtterance();
// Set the text.
msg.text = text;
// Set the attributes.
//msg.volume = 0.5;
//msg.rate = 1.1;
//msg.pitch = 1.0;
// If a voice has been selected, find the voice and set the
// utterance instance's voice attribute.
//if (voiceSelect.value) {
//msg.voice = speechSynthesis.getVoices().filter(function(voice) { return voice.name == voiceSelect.value; })[0];
//}
console.log(isChinese(text));
if (isChinese(text)==false) {
//msg.voice = voices[19]
//msg.lang = "en-US"
} else {
//msg.voice = voices[57] //alex 19 ting-ting 57
//msg.lang = "zh-CN"
}
console.log(msg)
// Queue this utterance.
window.speechSynthesis.speak(msg);
}
function replaceKeyword(string, word) {
var dmp = new diff_match_patch();
dmp.Diff_Timeout = parseFloat(100);
dmp.Diff_EditCost = parseFloat(4);
var max = 0
var max_word
var textes = string.split(" ");
textes.forEach(function(text, i) {
var diff = dmp.diff_main(word, text);
var counter = 0
for(let i=0; i
max) {
max = counter
max_word = text
}
});
return string.replace(max_word, "***")
}
const handleUpload = event => {
const files = event.target.files
const formData = new FormData()
const newFile = new window.File([files[0]], files[0].name+'.'+sender_id, {type: files[0].type})
//formData.append('file', files[0])
formData.append('file', newFile)
console.log(newFile.name)
$(".uploadform").remove();
// 发送跨域请求
//fetch("https://api.example.com/data", {
// mode: "cors",
// headers: {
// "Content-Type": "application/json",
// "Access-Control-Allow-Origin": "https://example.com",
// },
//})
setUserResponse("send file "+files[0].name);
fetch('/upload', {
method: 'POST',
body: formData
})
.then(response => response.json())
.then(data => {
console.log(data)
console.log(data['code'])
if (data['code'] == 0) {
send("send file");
} else {
setUserResponse("failed sending file");
}
})
.catch(error => {
console.error(error)
})
}
function startTyping(str, msgnode, end) {
if (str.length > 20) {
startTypingSentence(str, msgnode, end)
return
}
let str_ = ''
let i = 0
let content = msgnode
let timer = setInterval(()=> {
if(str_.length {
if(str_.length {
if(i < texts.length) {
str_ = texts[i++]
//content.innerHTML = ' ' + str_
content.innerHTML = '' + str_ + '
'
} else {
clearInterval(timer)
//content.innerHTML = str_
setTimeout(function () {
content.innerHTML = end
}, 1500);
}
},1500)
}
function getUUID(){
var rn = Number(Math.random().toString().substr(2,10) + Date.now()).toString(36)
return rn.substr(2, 8)
}
function getACookie(cookieName) {
let nameOfCookie = cookieName + '=';
let cookieDecoded = decodeURIComponent(document.cookie);
let cookieArray = cookieDecoded.split(';');
for (let i = 0; i < cookieArray.length; i++) {
let cIndex = cookieArray[i];
while (cIndex.charAt(0) == ' ') {
cIndex = cIndex.substring(1);
}
if (cIndex.indexOf(nameOfCookie) == 0) {
return cIndex.substring(nameOfCookie.length, cIndex.length);
}
}
return '';
}